Overview
GR-CITRUS can be run using the Ruby language. This section is dedicated to Ruby beginners. We’ll learn Ruby fundamentals while running a simple program.
Click on the following links for more details about the Ruby language. I have basically used the C language for programming up to this point, so I will focus on describing the differences between Ruby and C, and the benefits of Ruby.
- Help and documentation for the Ruby 2.3.0 programming language (in English)
- Ruby Language Summary
- Ruby 2.3.0 Reference Manual
Preparation
Hardware
You will need a GR-CITRUS board and a USB cable (Micro B type).
Software
We will be using Rubic for this project, so please familiarize yourself with the tool by referencing the Starting out with Rubic (Ruby Version) project page.
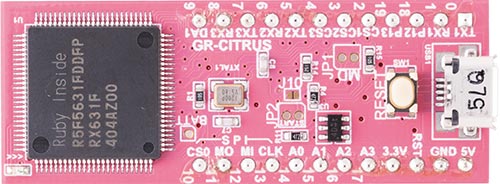
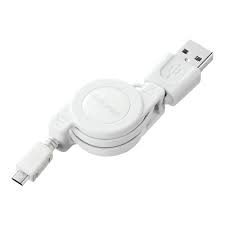
Display
First, we start with Hello World!
The serial class print is used to send a message to the connected PC when confirming the status of the program flow. Note that puts and p(“object”), both used frequently in Ruby, will generate syntax errors. Also, new generates a serial class instance, and argument 0 indicates use of USB.
usb = Serial.new(0)
usb.print("Hello")
(Result)
Hello
printIn
To insert a line feed, use printIn.
usb = Serial.new(0)
usb.println("Hello")
usb.print("Hello")
(Result)
Hello
Hello
Variables
First, we make the program carry out an operation. An operation requires a variable. Ruby does not use the kind of declarations you see in the C language. But you have to recognize it if you are dealing with numbers or characters.
How to Handle Numerical Values
Declarations like those used in the C language are not necessary. However, when displaying the variable with print, because the argument is a character string, you will need to convert the string from numerical values using the to_s method.
usb = Serial.new(0)
a=7
usb.println(a.to_s) # integer to string
a+=1
usb.println(a.to_s)
(Result)
7
8
How to Handle Character Strings
To put a character string in a variable, simply enclose the string in double quotation marks.
usb = Serial.new(0)
a="123"
usb.println(a)
a+="abc"
usb.println(a)
(Result)
123
123abc
To include double quotations, enclose the entire string in single quotation marks.
usb = Serial.new(0)
a = '"a"'
usb.println(a.to_s)
(Result)
"a"
Converting a Character String to a Numerical Value
To convert a character string to a numerical value, use the to_i method for integers and to_f method for floating points.
usb = Serial.new(0)
a ="123.001"
usb.println(a)
b = a.to_i + 456.12
usb.println(b.to_s)
b = a.to_f + 456.12
usb.println(b.to_s)
(Result)
123.001
579.12
579.121
String Manipulation
Ruby string manipulation is really powerful. Here is part of a sample to give you an idea.
usb = Serial.new(0)
a ="aabbccddeeaabbccddee"
usb.println(a)
usb.println(a.length.to_s) #length
usb.println(a.include?("ee").to_s) #include
usb.println(a.upcase) #upcase
usb.println(a.downcase) #downcase
usb.println(a.gsub("a","A")) #gsub (replace)
(Result)
aabbccddeeaabbccddee
20
true
AABBCCDDEEAABBCCDDEE
aabbccddeeaabbccddee
AAbbccddeeAAbbccddee
Arrays
How to Create an Array
Arrays are created without declarations. The index starts from 0, the same as with the C language.
usb = Serial.new(0)
a = [1,2,3,4]
usb.println(a[2].to_s)
(Result)
3
Elements
Both numerical values and character strings can be elements, as well as a mix of both.
usb = Serial.new(0)
a = [1, "def", 3.14]
usb.println(a[1].to_s)
(Result)
def
Adding or Joining Elements
Manipulating arrays in Ruby is really easy. This example shows how to add and join using unshift, push and concat.
usb = Serial.new(0)
a = ["pen"]
a.unshift("apple")
a.each do |n|
usb.print(n)
usb.print(" ")
end
usb.println()
b = ["pen"]
b.push("pineapple")
b.each do |n|
usb.print(n)
usb.print(" ")
end
usb.println()
b.concat(a)
b.each do |n|
usb.print(n)
usb.print(" ")
end
(Result)
apple pen
pen pineapple
pen pineapple apple pen
each
The each method retrieves the array in the specified order.
usb = Serial.new(0)
a = [1, 2.3, "abc", [1,2]]
a.each do |n|
usb.println(n.to_s)
end
(Result)
1
2.3
abc
[1,2]
Loop
This is equivalent to for and while in the C language, and can be used in Ruby as well.
while
This is an example of the well-used while. It is repeated if the conditional statement is true.
usb = Serial.new(0)
a = 5
while a > 0 do
usb.println(a.to_s)
a = a - 1
end
(Result)
5
4
3
2
1
times
This runs the operation for the specified number of times. If you don’t want to use variable a, you can eliminate |a|.
usb = Serial.new(0)
5.times do |a|
usb.println(a.to_s)
end
(Result)
0
1
2
3
4
for
This runs part of the range object (from 1…3). More specifically, it runs the partial operation the number of times described in the each method (described later) to retrieve the array.
usb = Serial.new(0)
for a in 1..3 do
usb.println(a.to_s)
end
(Result)
0
1
2
3